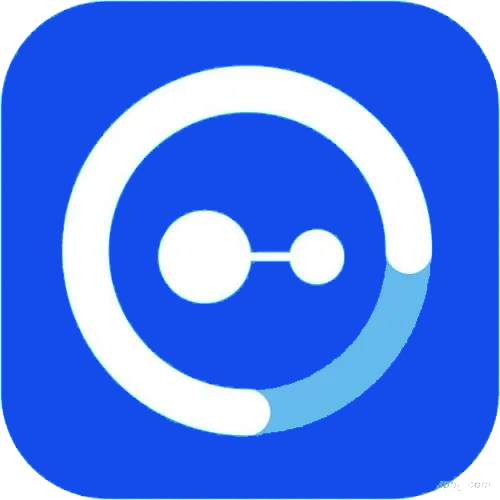
问答社区
BeautifulSoup是Python的一个库,用于从HTML和XML文件中提取数据
BeautifulSoup是Python的一个库,用于从HTML和XML文件中提取数据。它与请求库(如`requests`)结合使用,可以方便地爬取网页内容并解析HTML结构。以下是如何使用BeautifulSoup库的基本步骤:
### 1. 安装BeautifulSoup和requests库
首先,你需要安装BeautifulSoup和requests库。你可以使用pip来安装:
```bash
pip install beautifulsoup4 requests
```
### 2. 发送HTTP请求获取网页内容
使用`requests`库发送HTTP请求,获取目标网页的HTML内容:
```python
import requests
url = 'http://example.com'
response = requests.get(url)
html_content = response.text
```
### 3. 解析HTML内容
使用BeautifulSoup解析获取到的HTML内容:
```python
from bs4 import BeautifulSoup
soup = BeautifulSoup(html_content, 'html.parser')
```
### 4. 查找和提取数据
BeautifulSoup提供了多种方法来查找和提取HTML中的数据。以下是一些常用的方法:
- `find()`: 查找第一个匹配的元素
- `find_all()`: 查找所有匹配的元素
- `select()`: 使用CSS选择器查找元素
#### 示例:查找所有图片
假设你想查找网页中的所有图片(`<img>`标签),并提取图片的URL:
```python
images = soup.find_all('img')
for img in images:
img_url = img.get('src')
print(img_url)
```
#### 示例:查找特定元素
假设你想查找网页中的所有标题(`<h1>`标签):
```python
titles = soup.find_all('h1')
for title in titles:
print(title.text)
```
#### 示例:使用CSS选择器
假设你想查找所有带有特定类名的段落(`<p>`标签):
```python
paragraphs = soup.select('p.classname')
for p in paragraphs:
print(p.text)
```
### 5. 处理和存储数据
你可以将提取的数据存储到文件或数据库中。例如,将数据保存到CSV文件:
```python
import csv
with open('data.csv', 'w', newline='') as file:
writer = csv.writer(file)
writer.writerow(['Title', 'Link']) # 写入表头
for title in titles:
writer.writerow([title.text, title.get('href')]) # 写入数据行
```
### 6. 完整示例
以下是一个完整的示例,展示如何使用BeautifulSoup和requests库爬取网页中的图片URL:
```python
import requests
from bs4 import BeautifulSoup
# 发送HTTP请求获取网页内容
url = 'http://example.com'
response = requests.get(url)
html_content = response.text
# 解析HTML内容
soup = BeautifulSoup(html_content, 'html.parser')
# 查找所有图片并提取URL
images = soup.find_all('img')
for img in images:
img_url = img.get('src')
print(img_url)
```
通过这些步骤,你可以使用BeautifulSoup库轻松地解析HTML内容并提取所需的数据。